Given an array arr of integers, check if there exist two indices i and j such that :
- i != j
- 0 <= i, j < arr.length
- arr[i] == 2 * arr[j]
Example 1:
Input: arr = [10,2,5,3]
Output: true
Explanation: For i = 0 and j = 2, arr[i] == 10 == 2 * 5 == 2 * arr[j]
Example 2:
Input: arr = [3,1,7,11]
Output: false
Explanation: There is no i and j that satisfy the conditions.
Constraints:
- 2 <= arr.length <= 500
- -103 <= arr[i] <= 103
class Solution:
def checkIfExist(self, arr: List[int]) -> bool:
i = 0
j = 1
result = False
while i != len(arr) - 1:
if j != len(arr):
if arr[i] == arr[j] * 2 or arr[i] * 2 == arr[j]:
result = True
break
else:
j += 1
else:
i += 1
j = i+1
return result
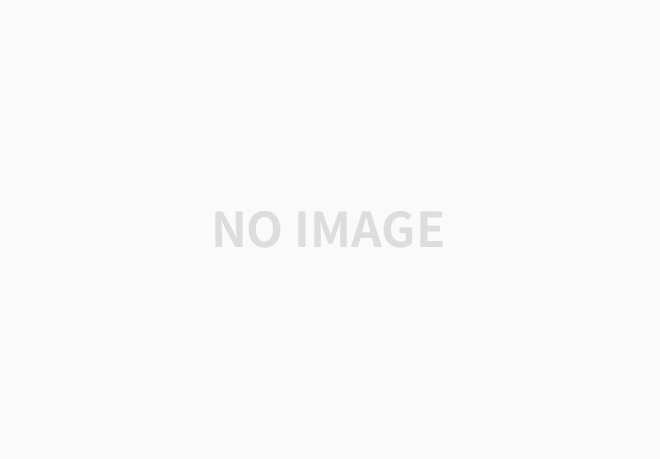
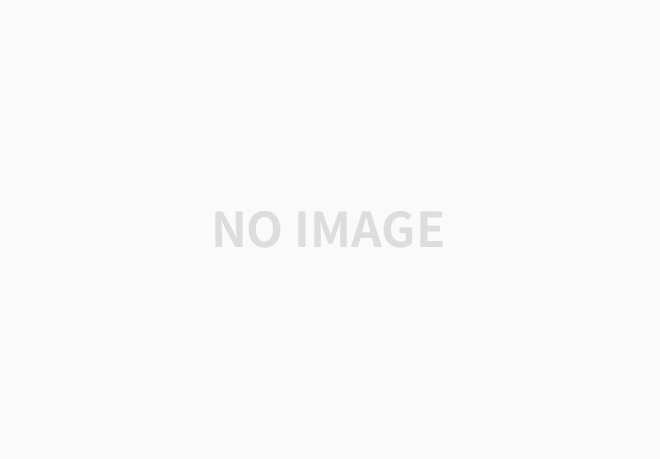
투포인터 방법을 공부하고 적용해보았습니다.(투포인터라고 하기 애매합니다.)
결과는 속도는 느리지만 메모리는 더 적게 사용했습니다.
바이너리 서치 알고리즘으로 푸는 방법도 있겠다는 생각도 되네요
'알고리즘 > 배열(array)' 카테고리의 다른 글
Replace Elements with Greatest Element on Right Side python (0) | 2023.07.03 |
---|---|
Valid Mountain Array python (0) | 2023.07.02 |
Remove Duplicates from Sorted Array python (0) | 2023.06.29 |
Merge Sorted Array python (0) | 2023.06.26 |
Duplicate Zeros python (0) | 2023.06.26 |